詳細資訊
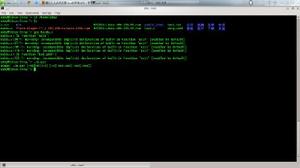
struct hostent:
h_name – 地址的正式名稱。
h_aliases – 空位元組-地址的預備名稱的指針。
h_addrtype –地址類型; 通常是AF_INET。
h_length – 地址的比特長度。
h_addr_list – 零位元組-主機網路地址指針。網路位元組順序。
h_addr - h_addr_list中的第一地址。
示例
#include
#include
#include
#include
#pragma comment(lib, "wininet.lib")
int main(int argc, char **argv)
{
//-----------------------------------------
// Declare and initialize variables
WSADATA wsaData;
int iResult;
DWORD dwError;
int i = 0;
struct hostent *remoteHost;
char *host_name;
struct in_addr addr;
char **pAlias;
// Validate the parameters
if (argc != 2) {
printf("usage: %s ipv4address\n", argv);
printf(" or\n");
printf(" %s hostname\n", argv);
printf("to return the host\n");
printf(" %s 127.0.0.1\n", argv);
printf("to return the IP addresses for a host\n");
printf(" %s www.tangzhigui.com \n", argv);
return 1;
}
// Initialize Winsock
iResult = www.qz888.com/ WSAStartup(MAKEWORD(2, 2), &wsaData);
if (iResult != 0) {
printf("WSAStartup failed: %d\n", iResult);
return 1;
}
host_name = argv;
// If the user input is an alpha name for the host, use gethostbyname()
// If not, get host by addr (assume IPv4)
if (isalpha(host_name)) { /* host address is a name */
printf("Calling gethostbyname with %s\n", host_name);
remoteHost = gethostbyname(host_name);
} else {
printf("Calling gethostbyaddr with %s\n", host_name);
addr.s_addr = inet_addr(host_name);
if (addr.s_addr = www.qz888.com = INADDR_NONE) {
printf("The IPv4 address entered must be a legal address\n");
return 1;
} else
remoteHost = gethostbyaddr((char *) &addr, 4, AF_INET);
}
if (remoteHost == NULL) {
dwError = WSAGetLastError();
if (dwError != 0) {
if (dwError == WSAHOST_NOT_FOUND) {
printf("Host not found\n");
return 1;
} else if (dwError == WSANO_DATA) {
printf("No data record found\n");
return 1;
} else {
printf("Function failed with error: %ld\n", dwError);
return 1;
}
}
} else {
printf("Function returned:\n");
printf("\tOfficial name: %s\n", remoteHost->h_name);
for (pAlias = remoteHost->h_aliases; *pAlias != 0; pAlias++) {
printf("\tAlternate name #%d: %s\n", ++i, *pAlias);
}
printf("\tAddress type: ");
switch (remoteHost->h_addrtype) {
case AF_INET:
printf("AF_INET\n");
break;
case AF_INET6:
printf("AF_INET6\n");
break;
case AF_NETBIOS:
printf("AF_NETBIOS\n");
break;
default:
printf(" %d\n", remoteHost->h_addrtype);
break;
}
printf("\tAddress length: %d\n", remoteHost->h_length);
if (remoteHost->h_addrtype == AF_INET) {
while (remoteHost->h_addr_list[i] != 0) {
addr.s_addr = *(u_long *) remoteHost->h_addr_list[i++];
printf("\tIPv4 Address #%d: %s\n", i, inet_ntoa(addr));
}
} else if (remoteHost->h_addrtype == AF_INET6)
printf("\tRemotehost is an IPv6 address\n");
}
return 0;
}